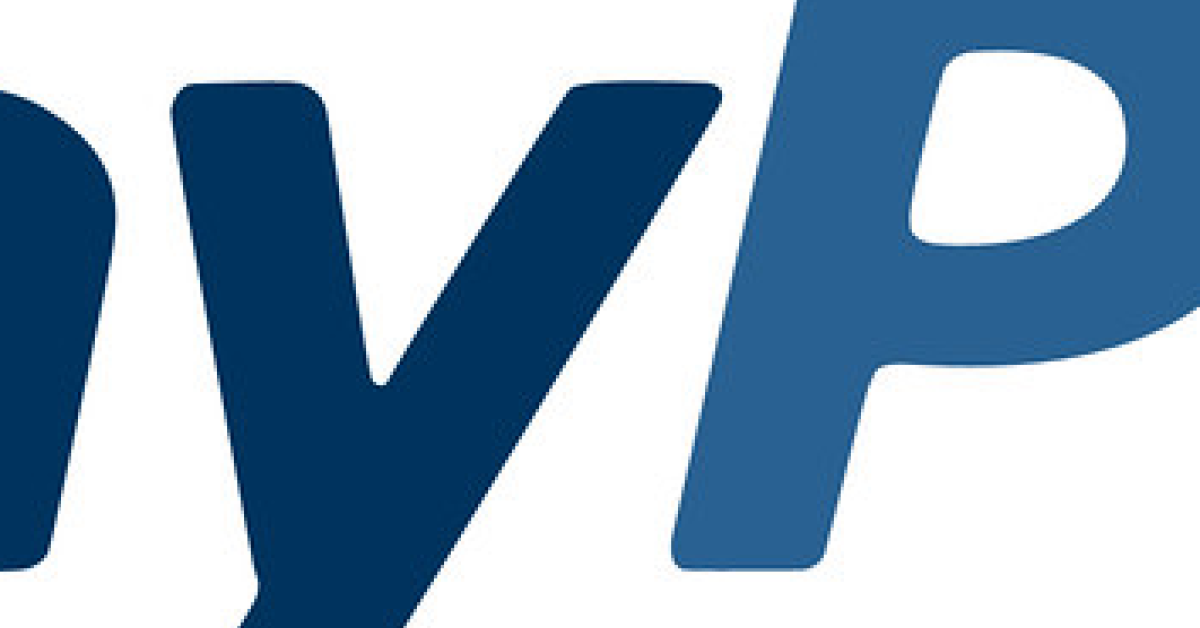
I encountered the frustrating issue of PayPal being unable to process payments and sought to understand the causes and potential solutions.
Recently, Fortect has become increasingly popular as a reliable and efficient way to address a wide range of PC issues. It's particularly favored for its user-friendly approach to diagnosing and fixing problems that can hinder a computer's performance, from system errors and malware to registry issues.
- Download and Install: Download Fortect from its official website by clicking here, and install it on your PC.
- Run a Scan and Review Results: Launch Fortect, conduct a system scan to identify issues, and review the scan results which detail the problems affecting your PC's performance.
- Repair and Optimize: Use Fortect's repair feature to fix the identified issues. For comprehensive repair options, consider subscribing to a premium plan. After repairing, the tool also aids in optimizing your PC for improved performance.
Troubleshooting PayPal Payment Errors
If you’re experiencing issues with PayPal and receiving an “Unable to Process Payment” error, follow these troubleshooting steps:
1. Check your payment details: Ensure that your credit card or bank account information is entered correctly. Double-check the numbers, expiry date, and CVV code.
2. Clear your browser cache: In your web browser settings, clear your cache and cookies. This can resolve any temporary issues that may be causing the error.
3. Update your browser: Make sure you’re using the latest version of your web browser, such as Google Chrome or Firefox. Go to the browser’s settings or help menu to check for updates.
4. Disable browser extensions: Some browser extensions or add-ons can interfere with PayPal’s payment process. Temporarily disable any extensions and try the payment again.
5. Try a different device: If you’re using PayPal on a mobile device or tablet, try switching to a different device or using the PayPal mobile app. Ensure you have a stable internet connection.
6. Contact PayPal support: If the issue persists, reach out to PayPal’s customer support for further assistance. Provide them with the error message and any relevant information about your transaction.
Alternative Solutions for PayPal Payment Issues
- Microsoft Payment Gateway: Explore using Microsoft’s own payment gateway as an alternative solution to overcome PayPal payment issues.
- Third-party Payment Gateways: Consider using alternative payment gateways such as Stripe or Square, which offer reliable payment processing services.
- Bank Transfer: In cases where PayPal is unable to process payments, consider bank transfers as a secure alternative to complete transactions.
- Credit/Debit Card Payments: Encourage customers to opt for credit or debit card payments as an alternative when PayPal encounters payment processing issues.
- Mobile Payment Apps: Suggest using mobile payment apps like Apple Pay or Google Pay as a convenient alternative for users facing PayPal payment issues.
- PayPal Express Checkout: Utilize PayPal Express Checkout as an alternative feature within PayPal’s services, which may help resolve payment errors.
Resolving Common PayPal Payment Problems
If you are experiencing the “PayPal Unable to Process Payment” error, there are a few common solutions you can try. First, ensure that you have a stable internet connection and that your device’s software is up to date. Clearing your browser’s cache and cookies can also help resolve the issue. Additionally, double-check that your PayPal account has sufficient funds or a valid payment method attached.
If the problem persists, contacting PayPal customer support for further assistance is recommended.
python
import paypal
def process_payment(payment_data):
try:
paypal.process_payment(payment_data)
print("Payment processed successfully!")
except paypal.InsufficientFundsError:
print("Unable to process payment. Insufficient funds.")
except paypal.PaymentMethodError:
print("Unable to process payment. Issue with payment method.")
except paypal.AccountLimitationsError:
print("Unable to process payment. Account limitations.")
except paypal.TechnicalError:
print("Unable to process payment. Technical issues.")
except Exception as e:
print("An unexpected error occurred:", str(e))
# Usage example:
payment = {
"amount": 100.0,
"payment_method": "credit_card",
"credit_card_number": "1234567890123456",
"expiration_date": "12/23",
"cvv": "123"
}
process_payment(payment)
Please note that this code is purely for illustrative purposes and does not interact with PayPal’s actual API. The `process_payment` function simulates a payment processing operation and handles potential errors using custom exceptions. You would need to replace the `paypal` module and its exception classes with the corresponding PayPal API or SDK in a real implementation.
Creating a New Account in PayPal Sandbox for Testing Purposes
Creating a New Account in PayPal Sandbox for Testing Purposes | |
---|---|
Error Title: | PayPal Unable to Process Payment Error |
Article Title: | PayPal Unable to Process Payment Error – Troubleshooting and Solutions |
Table of Contents: |
|
Section 3: Creating a New Account in PayPal Sandbox | |
Step | Description |
1 | Go to the PayPal Developer website |
2 | Click on the “Dashboard” tab |
3 | Under the “Sandbox” section, click on “Accounts” |
4 | Click on “Create Account” |
5 | Select the account type (Business or Personal) |
6 | Fill in the required details for the account |
7 | Click on “Create Account” |
8 | The new account will be created and displayed in the list of sandbox accounts |
